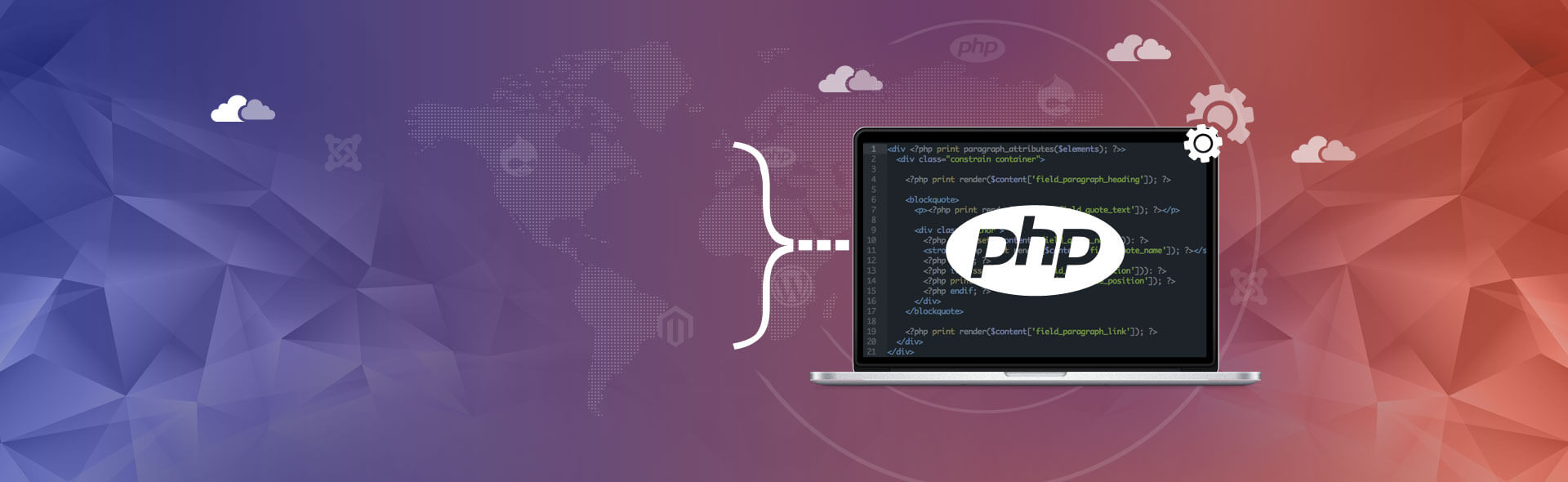
1. What is PHP and why is it used in web development?
Answer: PHP (Hypertext Preprocessor) is a server-side scripting language designed specifically for web development. It is embedded within HTML code and allows developers to create dynamic and interactive websites. PHP can interact with databases, handle forms, and manage sessions, making it a critical tool for building web applications.
2. How do you define variables in PHP?
Answer: In PHP, variables are defined by starting with a dollar sign ($
) followed by the variable name. PHP is loosely typed, meaning variables don’t need to be declared with a specific type. Example: $name = "John";
defines a variable $name
with the value “John”. PHP variables can hold strings, integers, arrays, and objects.
3. What are the different data types supported by PHP?
Answer: PHP supports several data types:
Scalar types: Integer, Float (double), String, Boolean
Compound types: Arrays, Objects
Special types: NULL, Resource Data types are determined dynamically, meaning they can change during execution based on the assigned value.
4. What is the difference between include()
and require()
in PHP?
Answer: Both include()
and require()
are used to include the contents of a PHP file in another file. The key difference is in error handling:
include()
: Generates a warning but continues script execution if the file is not found.
require()
: Generates a fatal error and stops the script if the file is not found. Use require()
when the file is crucial for the application to run, and include()
when the file is optional.
5. What is the purpose of the $_POST
and $_GET
superglobals in PHP?
Answer: Both $_POST
and $_GET
are superglobal arrays used to collect form data.$_GET
: Collects data sent through the URL, typically used for simple form submissions or when the data needs to be visible in the URL.$_POST
: Collects data sent via HTTP POST method, used for submitting sensitive data (like passwords) and larger amounts of data. Example: $_GET['name']
retrieves the value from a URL parameter like ?name=John
.
6. Explain the concept of a session in PHP. How is it different from cookies?
Answer: A session in PHP is a way to store data across multiple pages during a user’s visit. When a session is started with session_start()
, PHP creates a unique session ID, and session variables can be stored on the server. Sessions are useful for storing login data or user preferences.
Cookies, on the other hand, are small pieces of data stored on the client-side (in the browser) and can expire or be deleted.
Key difference: Sessions are more secure because the data is stored on the server, while cookies store data on the client-side.
7. What are arrays in PHP? Can you explain the difference between indexed and associative arrays?
Answer: Arrays in PHP are variables that can hold multiple values. PHP supports two main types of arrays:
Indexed arrays: Arrays with numeric indexes (0, 1, 2, etc.). Example: $colors = ["Red", "Blue", "Green"];
Associative arrays: Arrays where keys are assigned manually, often strings. Example: $person = ["name" => "John", "age" => 30];
Arrays are fundamental for organizing and managing groups of data efficiently.
8. What is the difference between ==
and ===
in PHP?
Answer: In PHP:
==
is the equality operator, which checks if the values of two variables are equal, after performing type conversion if necessary.
===
is the identical operator, which checks whether two variables are equal in both value and type. For example, 5 == "5"
returns true, but 5 === "5"
returns false because one is an integer and the other is a string.
9. How do you handle errors in PHP?
Answer: PHP provides multiple ways to handle errors:
Error reporting: Use error_reporting(E_ALL)
to report all errors or specific types like E_WARNING
, E_NOTICE
, etc.
Try-catch blocks: In PHP 5 and later, exceptions can be caught using try
and catch
blocks for better error handling and debugging.
Custom error handlers: You can set a custom error handler using set_error_handler()
for more control over error handling.
10. What is the isset()
function in PHP?
Answer: The isset()
function checks whether a variable is set and is not NULL
. It returns true
if the variable exists and has a non-NULL
value, and false
otherwise. This function is commonly used to check whether form data or variables are defined before trying to use them, helping to prevent errors.
Comments (0)
No comments yet. Be the first to comment!