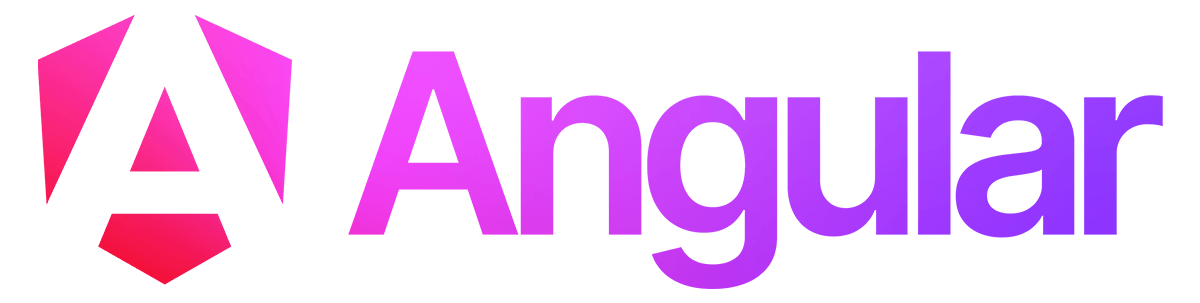
1. What is the difference between ngOnInit
and constructor
in Angular?
Answer: Both ngOnInit
and the constructor
are part of Angular’s component lifecycle. The constructor
is a TypeScript feature that initializes class members, and it is called when the class is instantiated. However, it is used for simple initialization and does not interact with Angular’s dependency injection system.
ngOnInit
, on the other hand, is a lifecycle hook that is called after Angular has initialized the component’s input properties. It’s the best place for component initialization, especially when you need to make API calls or interact with data-bound properties.
2. What is Dependency Injection in Angular?
Answer: Dependency Injection (DI) in Angular is a design pattern used to implement Inversion of Control (IoC), allowing Angular to manage the creation and management of objects or services. Instead of manually creating instances of services within a component or class, Angular automatically injects the required services, making code more modular, maintainable, and testable.
Angular’s DI system provides services to components and other services through constructors, and services can be injected into constructors by specifying them as parameters.
3. What are Angular Directives? Give examples.
Answer: Directives are Angular’s way of extending HTML with new functionality. They can manipulate the DOM by adding behavior to elements or controlling their visibility.
There are three types of directives in Angular:
Structural Directives: They change the structure of the DOM, such as *ngIf
, *ngFor
, and *ngSwitch
.
Attribute Directives: They change the appearance or behavior of an element, such as ngClass
, ngStyle
, and custom directives.
Component Directives: These are the building blocks of Angular applications, like <app-component>
.
4. What is Angular Change Detection?
Answer: Change detection is a mechanism in Angular that checks the state of the application and determines whether the view (UI) needs to be updated. Angular uses a dirty checking mechanism, where it compares the current value of a component’s properties with the previous ones to decide whether the DOM needs to be updated.
Angular’s change detection strategy can be controlled using ChangeDetectionStrategy.Default
(which checks all components) and ChangeDetectionStrategy.OnPush
(which checks only when inputs to a component change).
5. What are Observables in Angular and how are they used?
Answer: Observables are a core concept in Angular, coming from the RxJS library, and are used for asynchronous programming. Observables can handle asynchronous data streams, such as HTTP requests, user input events, or WebSocket connections.
In Angular, the HttpClient
service returns Observables for making HTTP requests, and you can subscribe to them to handle responses. Observables also provide powerful operators for filtering, transforming, and composing data streams, allowing for cleaner and more scalable code.
6. What is the purpose of ngFor
in Angular?
Answer: ngFor
is a structural directive in Angular used to iterate over a list of items in the DOM. It dynamically creates a template for each item in the array or list, and you can bind the template’s content to the current item in the loop.
Example usage:
<div *ngFor="let item of items">
{{ item.name }}
</div>
This will repeat the <div>
for each item
in the items
array.
7. What is a Service in Angular and how is it used?
Answer: A Service in Angular is a class that provides reusable logic, data management, and business logic across components. Services are typically used for handling data, HTTP requests, or implementing shared functions like authentication.
Services are injected into components via Angular’s Dependency Injection system, ensuring that the same instance of a service is used across the application. This improves code reusability and testability.
@Injectable({
providedIn: 'root',
})
export class MyService {
constructor(private http: HttpClient) {}
}
8. What is the difference between @Input()
and @Output()
decorators in Angular?
Answer:
@Input()
allows a parent component to pass data to a child component. It binds a property in the child component to a value in the parent component.
Example:
@Input() data: string;
@Output()
is used to send data from the child component to the parent component. It emits an event using an EventEmitter
, allowing the parent component to respond.
Example:
@Output() change = new EventEmitter<string>();
9. Explain Angular’s lifecycle hooks.
Answer: Angular components go through a series of lifecycle hooks that allow developers to perform actions at different points in the component’s lifecycle. Some key lifecycle hooks include:
ngOnChanges()
: Called when input properties change.ngOnInit()
: Called once the component’s data-bound properties are initialized.ngDoCheck()
: Called during every change detection cycle.ngAfterViewInit()
: Called after the component’s view and its child views are initialized.ngAfterContentInit()
: Called after content projected into the component is initialized.ngOnDestroy()
: Called just before the component is destroyed.
Each of these hooks gives you control over the behavior of your component during its lifecycle.
10. What is RxJS
and how does it relate to Angular?
Answer: RxJS
(Reactive Extensions for JavaScript) is a library for handling asynchronous events and data streams using Observables. It plays a crucial role in Angular, especially when dealing with HTTP requests, user events, or any asynchronous data.
Angular uses RxJS to handle operations such as fetching data, chaining multiple operations together, and managing async data streams. With RxJS operators like map
, filter
, merge
, and switchMap
, developers can compose complex logic in a clean and maintainable way.
11. What is Angular CLI and how is it used?
Answer: Angular CLI (Command Line Interface) is a powerful tool used to simplify the development process. It helps developers scaffold Angular projects, generate components, services, directives, and other Angular constructs, run unit tests, and build the production-ready application.
Some common Angular CLI commands include:
ng new my-app
: Creates a new Angular project.ng generate component component-name
: Generates a new component.ng serve
: Starts the development server.ng build
: Builds the project for production.
12. How does Angular handle form validation?
Answer: Angular provides two ways to manage forms and validation: Template-driven forms and Reactive forms.
a. Template-driven forms are easier to implement and work with the HTML form elements directly, using directives like ngModel
and built-in validation rules like required
, minlength
, and maxlength
.Example:
<form #myForm="ngForm">
<input type="text" name="name" ngModel required>
</form>
b. Reactive forms offer a more programmatic approach, where form controls and validation logic are defined explicitly in the component class. They provide more control over the form’s state and validation.
Example:
this.form = this.fb.group({
name: ['', [Validators.required, Validators.minLength(3)]],
});
13. What is lazy loading in Angular?
Answer: Lazy loading is a technique used in Angular to load modules only when they are needed, instead of loading the entire application upfront. This improves the application’s initial loading time and performance.
By using Angular’s loadChildren
property in routing configuration, modules can be loaded dynamically.
Example:
const routes: Routes = [
{
path: 'feature',
loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule)
}
];
14. What are Angular pipes and how do they work?
Answer: Pipes are used to transform data in the template. They take input values, transform them, and return the transformed result. Angular comes with built-in pipes like date
, currency
, and uppercase
. You can also create custom pipes to meet specific needs.
Example of using the date
pipe:
<p>{{ currentDate | date:’short’ }}</p>
To create a custom pipe, you can implement the PipeTransform
interface:
@Pipe({ name: 'customPipe' })
export class CustomPipe implements PipeTransform {
transform(value: string): string {
return value.toUpperCase();
}
}
Comments (0)
No comments yet. Be the first to comment!