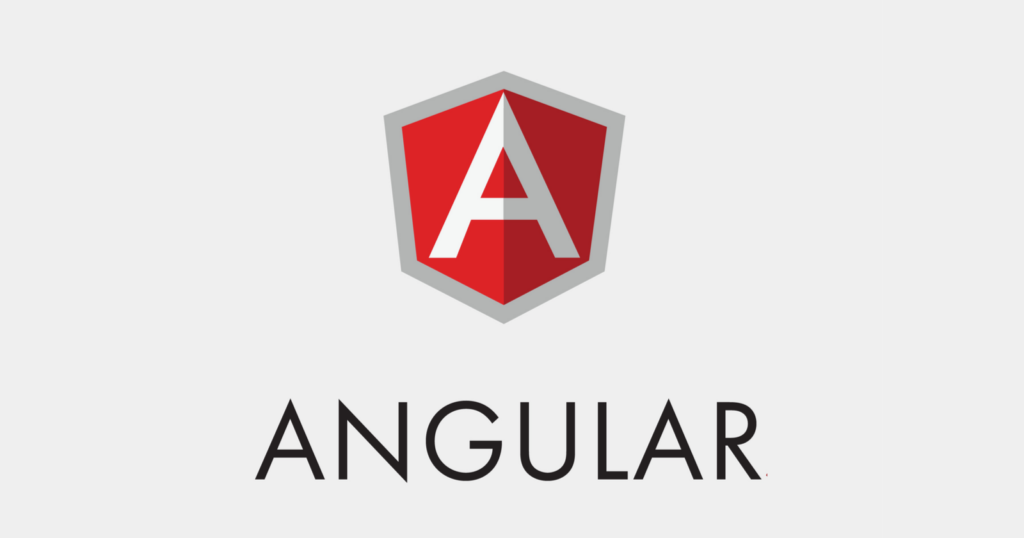
1. What is Angular?
Answer:
Angular is a popular open-source framework that is developed and maintained by Google to build dynamic single-page web applications. It is made up of TypeScript and helps you achieve things such as two-way data binding, dependency injection, modularity, and component-based architecture to build scalable and maintainable web applications.
2. What is a Component in Angular?
Answer:
Component is considered the basic building block of an Angular application which modulates a part of the UI, encapsulating them—e.g., HTML template, CSS, and their behaviors. Every component in Angular is, at least, a TypeScript class, and HTML template and an optional CSS. Components also can be the designer who, by their highly reusable nature, breaks down the complete UI into composable bits.
3. What is Two-Way Data Binding in Angular?
Answer:
The two-way binding feature in Angular ensures the automatic synchronization of data between the model (the component class) and the view (the template). Since if data is changed in the view, it is reflected in the model; hence, changes remain synchronized always. A polite convention uses [(ngModel)] for this.
4. What is Dependency Injection (DI) in Angular?
Answer:
Dependency injection is one of the features of Angular to assign dependent objects and other resources (commonly services) to an application component for further use. Central to Angular makes an Injector known as a built-in service provider. Providers are used to inject templates, components, and services.
5. What is a Service in Angular?
Answer:
In that class developed from scratch, services are merely utility classes that deliver shared data and functionality. Usually, they are used for HTTP requests, business logic, or shared data among components. They are then injected into components through Angular’s Dependency Injection (DI) system.
6. What is Routing in Angular?
Answer:
Routing in Angular is the trigger that takes a user from one view to another as per the action the user takes on the navigational element. The roles defined in Angular for routing are meant to help the developer define routes where components are displayed. This is a modern type of lazy loading, and guards could be used to implement this process.
7. What is the Difference Between ngOnInit()
and the Constructor in Angular?
Answer:
The constructor is a type of function that gets called when a class instance is being created. It is typically used for initialization purposes and is the first one to get called. In contrast, ngOnInit() is defined from the interfaces for lifecycle hooks that are called once the component has finished initializing all the “@Input
” properties with the corresponding trait values. It is generally used to do further object creation or data fetching in a component.
8. What is the Purpose of ngFor
in Angular?
Answer:
ngFor is a structural directive that can iterate over an array or collection enabling the dynamic rendering of items in any view. This makes it dramatically easy to render lists in the templates. A simple application of which might be iterating the items from a component’s array inside an HTML template.
9. What is Angular CLI?
Answer:
The Angular Command Line Interface, or Angular CLI, is a powerful and easy-to-navigate tool to help automate the creation, building, and managing of Angular applications. It speeds up development by providing commands to generate new components, services, modules, etc. It supports many other features like testing, linting, and deployment as well.
10. What are Directives in Angular?
Answer:
Directives in Angular are entities applied on elements in the DOM to modify its behavior or appearance. These are of three types:
Structural directives (like *ngIf, *ngFor) alter the structure of the DOM.
Attribute directives (like ngClass, ngStyle) change or tweak appearance and behavior of the elements in the DOM.
Custom directives can be made after they extend the functionalities of the Angular framework.
11. What is Angular Module?
Answer:
Modules in Angular refer to a system to organize related components, such as services, directives, pipes, and components, into a file or a folder. An Angular application includes at least one module, called the AppModule by convention. Modules serve as a means to organize our application, enabling developers to import them, and in return, they can export select features for other reuse.
Example:
// app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
12. What are Observables in Angular?
Answer:
Observables handle asynchronous data in Angular, allowing for subscriptions to data streams and reaction events such as HTTP requests, user inputs, or other async operations. RxJS Library provides observables, which Angular comes along with.
Example:
import { Observable } from 'rxjs';
// A simple observable example
const dataObservable = new Observable(observer => {
observer.next('Hello');
observer.next('World');
observer.complete();
});
// Subscribing to the observable
dataObservable.subscribe({
next: value => console.log(value),
complete: () => console.log('Completed')
});
Output:
Hello
World
Completed
13. What is ngIf
and How Does It Work?
Answer:
ngIf in Angular is a structural directive that conditionally includes or excludes elements from the DOM. The element gets added to the DOM when the condition is true in the ngIf expression; otherwise, it is removed.
Example:
<div *ngIf="isVisible">This element is visible</div>
export class AppComponent {
isVisible = true; // Change this to false to hide the element
}
If isVisible
is true
, the element will be displayed. If it’s false
, it will be removed from the DOM.
14. What is ngFor
and How Does It Work?
Answer:
The ngFor is a structural directive in Angular to loop over each item in a collection, possibly an array of items. It is used frequently to iterate through an array of items and display those on the template.
Example:
<ul>
<li *ngFor="let item of items">{{ item }}</li>
</ul>
export class AppComponent {
items = ['Apple', 'Banana', 'Cherry']; // Array to loop through
}
This will output:
nginxCopyApple
Banana
Cherry
15. What is the Difference Between const
and let
in Angular?
Answer:
const is initially used for declaring variables that are not to be reassigned. Once you define it, you cannot replace the value.
let defines a variable that can be changed throughout the execution of the program.
Example:
const PI = 3.14;
PI = 3.1415; // This will give an error
let radius = 5;
radius = 6; // This is allowed
16. What are Pipes in Angular?
Pipes in Angular are used mainly for data transformation at the template level. This is helpful in formatting data for display when modifying the actual value at the same time. Several inbuilt pipes are offered by Angular, such as date, currency, uppercase, lowercase and so on.
Example:
<!-- Date Pipe -->
<p>{{ today | date:'short' }}</p>
<!-- Uppercase Pipe -->
<p>{{ 'hello' | uppercase }}</p>
export class AppComponent {
today = new Date(); // Will display today's date
}
This will output:
02/24/2025
HELLO
17. What is the async
Pipe in Angular?
Answer:
It is an inbuilt Angular pipe that allows us to unwrap the async Observables or Promises easily in the template. The async pipe subscribes to the observable and destroys it when your component is destroyed.
Example:
<p>{{ observableData | async }}</p>
import { Observable, of } from 'rxjs';
export class AppComponent {
observableData: Observable<string> = of('Data from Observable');
}
This will output:
Data from Observable
18. What are Lifecycle Hooks in Angular?
Answer:
Lifecycle features are some of the ways designers can implement many things across the lifecycle of an Angular component. They help specify the behavior of a component over a given time interval and provide a way to interrupt the process. Lifecycle methods are:
ngOnInit()
ngOnChanges()
ngOnDestroy()
Example:
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-lifecycle',
template: '<p>Lifecycle hook example</p>',
})
export class LifecycleComponent implements OnInit {
ngOnInit() {
console.log('ngOnInit called');
}
}
The message ngOnInit called
will be logged to the console when the component is initialized.
19. How Does Angular Handle Forms?
Answer:
Angular possesses two types of forms: The template-driven and reactive forms.
The template-driven forms: Are template-centric and easier to handle.
Reactive forms: Are more flexible and provide more control. Perfect for a significant build.
Example of a Template-driven form:
<form #myForm="ngForm">
<input type="text" name="username" ngModel>
<button [disabled]="!myForm.valid">Submit</button>
</form>
20. What is Lazy Loading in Angular?
Answer:
Lazy loading is used to load Angular modules only when required – therefore cutting down on load time during the application start. It enhances or boosts performance by simply loading certain parts of an application when the user reaches them.
Example:
// app-routing.module.ts
const routes: Routes = [
{ path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) }
];
In this example, the FeatureModule will only be loaded when the user navigates to the /feature
route.
Comments (0)
No comments yet. Be the first to comment!